Blogs
Kubernetes Cheat Sheet
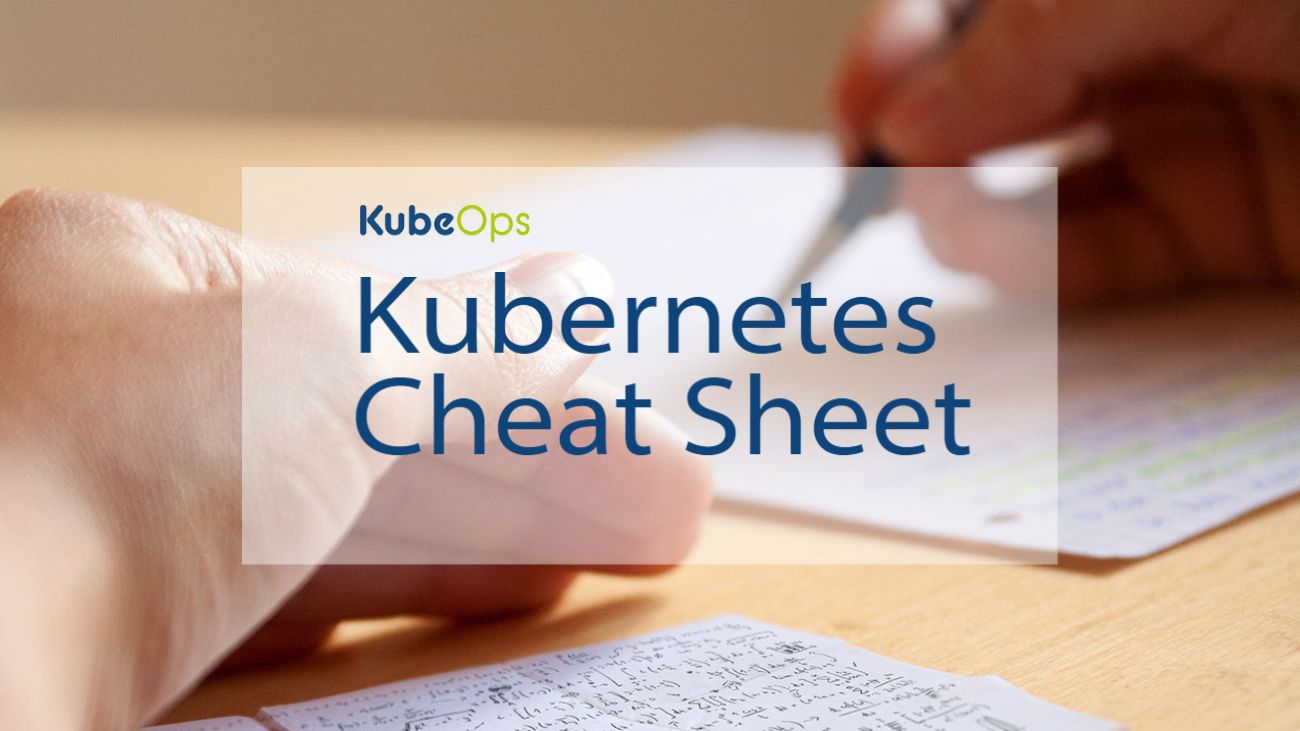
Kubernetes Objects
Node
List all nodes in the cluster
kubectl get nodes
Delete a node from the cluster
kubectl delete node <node name>
Show the metrics of a node
kubectl top node <node name>
Describe a node in detail
kubectl describe node <node name>
List all pods in a namespace, with more details
kubectl get node -o wide ¬-n <namespace>
Add an annotation to a node
kubectl annotate node <node name>
Add a label to given node
kubectl label node <node name> type=label
Show node labels
kubectl get nodes --show-labels
Show information about a node in yaml format
kubectl get node <node name> -o yaml
Show information about a node with the given label
kubectl get node --selector=<label>
Pod
List all the pods in a namespace
kubectl get pod -n <namespace>
List pods with more details
kubectl get pods -o wide
Show the detailed description of pod
kubectl describe pod <pod name>
Delete a pod with a file describing it
kubectl delete -f pod.yaml
Create a pod in a certain namespace
kubectl create pod <pod name> -n <namespace>
Create a pod from an image
kubectl run <pod name> --image=<image name>
Show logs of a pod
kubectl logs <pod name> -n <namespace>
Execute a command in a pod and get an interactive terminal
kubectl exec -it <pod name> -- <command>
List all running pods in a namespace
kubectl get pods --field-selector=status.phase=Running
Live monitor the state of a pod
kubectl get pod <pod name> --watch
Live monitor the state of all pod
kubectl get pod -A --watch
Show all pods in json format
kubectl get pods -o json
List all pods in all namespaces
kubectl get pods --all-namespaces
List all pods in all namespaces
kubectl get pods -A
Show metrics for a given pod
kubectl top pod <pod name>
Show metrics for a given pod and all its containers
kubectl top pod <pod name> --containers
View container logs of a pod (if it has more than one container)
kubectl logs <pod name> -c <container name>
Get the documentation for the pod manifest
kubectl explain pod
List pods with labels
kubectl get pods --show-labels
kubectl using a different kubeconfig
kubectl --kubeconfig=<path to config file> get pods
Get the initContainer status of a pod
kubectl get pod --template '{{.status.initContainerStatuses}}' <pod name>
Print a list of pods sorted by name
kubectl get pods --sort-by=.metadata.name
Deployment
Create a new deployment
kubectl create deployment <deployment name> --image=<image>
Create a new deployment from a manifest file
kubectl apply -f <deployment manifest>
List deployments
kubectl get deployment <deployment name>
Watch a specific deployment
kubectl get deployment <deployment name> --watch
List all deployments
kubectl get deployment -A
Show the detailed state of a deployment
kubectl describe deployment <deployment name>
Delete a deployment
kubectl delete deployment <deployment name>
Rolling update nginx of deployment
kubectl set image deployment/<deployment name> <container name>=<image>
Scale a deployment to 10 instances
kubectl scale --replicas=10 deployment/<deployment name>
Autoscale a deployment to stay near 80% cpu usage with the limitation of staying between 10-15 instances
kubectl autoscale deployment/<deployment name> --min=10 --max=15 --cpu-percent=80
Rolling back to the previous revision
kubectl rollout undo deployment/<deployment name>
Rolling back to a specific revision
kubectl rollout undo deployment/<deployment name> --to-revision=<revision name>
Check the rollout status of a deployment
kubectl rollout status deployment/<deployment name>
Show the revision history of a deployment
kubectl rollout history deployment/<deployment name>
Live edit a deployment
kubectl edit deployment/<deployment name>
Port forwarding of a deployment to a specific port
kubectl port-forward deployment/<pod name> <localhost port>:<pod port>
DeamonSets
List all the daemon sets in a namespace
kubectl get daemonset --namespace <namespace>
List all daemon sets in all namespaces
kubectl get daemonset --all-namespaces
Detailed information about a daemonset in yaml format
kubectl get daemonset <daemonset name> --namespace <namespace> -o yaml
Detailed information for a specific daemon set in a namespace
kubectl describe daemonset <daemonset name> --namespace <namespace>
Configmaps
Create configmap from a file
kubectl create configmap <configmap name> --from-file <configmap manifest>
Get more details about specific configmap
kubectl describe configmap <configmap name>
List all configmaps in a namespace
kubectl get configmap --namespace <namespace>
Get specific configmap in yaml format
kubectl get configmap <configmap name> -o yaml
List all configmaps in all namespaces in yaml format
kubectl get configmap --all-namespaces -o yaml
Services
List all services in a namespace
kubectl get services --namespace <namespace>
List the services in a namespace with additional information
kubcetl get service --namespace <namespace> -o wide
Show the detailed information of a service
kubectl describe service <service name>
Delete a particular service
kubectl delete service <service name>
Get the documentation for service manifests
kubectl explain service
Port forwarding a service
kubectl port-forward service/<service name> <localhost port>:<service-port>
Creates a Service of type nodeport with a mapping of post 8080 to 80
kubectl create service nodeport <service name> --tcp=8080:80
List services with their labels
kubect l get service --show-labels
Describe a service in yaml format
kubect l get service <service name> -o yaml
Service Account
List Service Accounts
kubectl get serviceaccounts
Detailed state of a service accounts
kubectl describe serviceaccount <serviceaccount name>
Replace a service account
kubectl replace serviceaccount <serviceaccount name>
Delete a service account
kubectl delete serviceaccount <serviceaccount name>
Live edit a service account
kubectl edit serviceaccount <serviceaccount name>
Secrets
kubectl describe secret/<secret name>
Show all secrets in a namespace
kubectl get secrets -n <namespace>
Describe a secret in more details
kubectl describe secret/<secret name>
List secrets in all namespaces
kubectl get secrets --all-namespaces
List secret in yaml format
kubectl get secret <secret name> -o yaml
Roles
List all roles in cluster
kubectl get roles --all-namespaces
List all clusterroles
kubectl get clusterroles
List all role bindings and clusterrolebindungs in the cluster
kubectl get (cluster)rolebinding --all-namespaces
Create the role “pod-reader” which can list and watch pods
kubectl create role pod-reader --verb=get --verb=list --verb=watch --resource=pods
Namespace
Create a namespace
kubectl create namespace <namespace name>
List all namespaces in a cluster
kubectl get namespaces
Get a namespace description in yaml format
kubectl get namespace <namespace name> -o yaml
Display detailed state of a namespace
kubectl describe namespace <namespace name>
Delete a namespace
kubectl delete namespace <namespace name>
Edit and update the definition of a namespace
kubectl edit namespace <namespace name>
Create a namespace using a yaml file
kubectl create -f namespace.yaml Create a namespace using a yaml file
Replicasets
List all replicasets in current namespace
kubectl get replicasets
Describe detailed a replicaset in detail
kubectl describe replicaset <replicaset name>
List ReplicaSets with more information
kubectl get replicaset -o wide
List ReplicaSet in yaml format
kubectl get replicaset <replicaset name> -o yaml
Get the documentation for the ReplicaSet manifest
kubectl explain replicaset
List all persistent volumes in a namespace
kubectl get persistantvolume -n <namespace>
Describe a persistent volume
kubectl describe persistantvolume <persistantvolume name>
Delete a persistent volume
kubectl delete persistantvolume < persistantvolume name>
List the storage classes in yaml format
kubectl get storageclass –o yaml
PersistentVolume Claim
List all persistent volume claims in a namespace
kubectl get persistentvolumeclaim <persistentvolumeclaim name>
Describe a persistent volume claim
kubectl describe persistentvolumeclaim <persistentvolumeclaim name>
Delete a persistent volume claim
kubectl delete persistentvolumeclaim <persistentvolumeclaim name>
List ingress resource
kubectl get ingress
List ingress resources in all namespaces
kubectl get ingress --all-namespaces
Get information about the ingress object
kubectl explain ingress
Get information about an ingress
kubectl describe ingress <ingress name>
List all ingress objects with their corresponding services
kubectl get ingress -o=custom-columns='NAME:.metadata.name,SVCs:..service.name'
Labels
List assigned labels on the node
kubectl get nodes --show-labels
List assigned labels on the pods
kubectl get pods --show-labels
Add label to node
kubectl label nodes <node name> <label>
Remove label from a node, same command but you see minus after the label name
kubectl label node <node name> <label>-
Set to the pod the label status and value unhealthy, overwriting any existing value
kubectl label --overwrite pods <pod name> status=unhealthy
Events
View all events in the cluster
kubectl get events --all-namespaces
List events in json format
kubectl get events -o json
Get events from of a pod
kubectl get events | grep <pod name>
This also shows events
kubectl describe pod <pod name>
List Events sorted by timestamp
kubectl get events --sort-by=.metadata.creationTimestamp
List all warning events
kubectl events --types=Warning
API Resources
Enumerates the resource types available
kubectl api-resources
List api group
kubectl api-versions
All namespaced resources
kubectl api-resources --namespaced=true
All non-namespaced resources
kubectl api-resources --namespaced=false
List all CRDs
kubectl get crd
List storageclasses
kubectl get storageclass
List the “healty” status of components
kubectl get componentstatuses
Themes
Logs & Conf files
Config folder
/etc/kubernetes/
Certificate files
/etc/kubernetes/pki/
Credentials to API server
/etc/kubernetes/kubelet.conf
Superuser credentials
/etc/kubernetes/admin.conf
kubectl config file
~/.kube/config
Kubernets working dir
/var/lib/kubelet/
Docker working dir
/var/lib/docker/, /var/log/containers/
Etcd working dir
/var/lib/etcd/
Network cni
/etc/cni/net.d/
Log files
/var/log/pods/
log in worker node
/var/log/kubelet.log, /var/log/kube-proxy.log
log in master node
kube-apiserver.log, kube-scheduler.log, kube-controller-manager.log
Quotas & limits
List Resource Quota
kubectl get resourcequota
List Limit Range
kubectl get limitrange
Customize the resource limit of a pod for CPU
kubectl set resources deployment nginx -c=nginx --limits=cpu=200m
Customize the resource limit of a pod for memory
kubectl set resources deployment nginx -c=nginx --limits=memory=512Mi
Annotations & Taints
Get annotations of deployment
kubectl describe deployment/<deployment name> | grep Annotations
Update annotations
kubectl annotate pods <pod name> <key>=<value>
Override annotation of a resource
kubectl annotate --overwrite <resource type> <resource name> <key>=<value>
Update the taints of a nodes
kubectl taint <node name> <taint name>
Common Metric Commands
Show metrics for a given node
kubectl top node <node name>
Show metrics for a given pod
kubectl top pod <pod name>
Show metrics for a given pod and all its containers
kubectl top pod <pod name> --containers
Common Log commands
Check logs of a pod
kubectl logs <pod name> --namespace <namespace>
Container logs in a pod (if more than one)
kubectl logs <pod name> <container name>
Logs from all containers in a pod
kubectl logs <pod name> --all-containers
Get logs from the last hour
kubectl logs --since=1h <pod name>
Display the most recent 20 lines of logs
kubectl logs --tail=20 <pod name>
Save the logs into a file
kubectl logs <pod name> <file name>
Get logs filtered by label
kubectl logs -l <label key>=<label value> --all-containers
Get logs from deployment
kubectl logs deployment/<deployment name>
Get logs from job
kubectl logs job/<job name>
Export logs to a file
kubectl logs <pod name> > <file name>
Security
List Network Policy
kubectl get networkpolicy
List certificates
kubectl get certificate
Show kubeconfig settings
kubectl config view
Get a List of contexts
kubectl config get-contexts
Display current context
kubectl config current-context
Set default context to <cluster name>
kubectl config use-context <cluster name>
Set namespace field for context
kubectl config set-context <context name> --namespace=<namespace>
Set cluster field for context
kubectl config set-context <context name> --cluster=<cluster name>
Set user field for context
kubectl config set-context <context name> --user=<username>
Maintenace
Mark the named node as unschedulable
kubectl cordon <node name>
Mark the named node as schedulable
kubectl uncordon <node name>
Drain the node in preparation for maintenance
kubectl drain <node name>
This will give you the pod CIDR addresses for each of the nodes in your cluster.
kubectl get nodes -o jsonpath='{.items[*].spec.podCIDR}'
Delete a resource matching a label
kubectl delete <resource type> -l <label key>=<label value>
Display addresses of master / services
kubectl cluster-info
Detailed info about the cluster state to stout
kubectl cluster-info dump
This will show pod network CIDR which used by kube-proxy
kubectl cluster-info dump | grep -m 1 cluster-cidr
Dump current cluster state to a given file
kubectl cluster-info dump --output-directory=/path/clusterinfo.txt
To get all objects of your cluster
kubectl get all --all-namespaces
List services, pods, nodes, deployments and replicasets
kubectl get svc, po, no, deploy, rs
Output yaml to a file, creating template
kubectl run <pod name> --image=nginx --dry-run=client -o yaml > pod1.yaml
Addendum: Docker
Install on Linux
Install docker in linux terminal
curl -sSL https://get.docker.com/ | sh
Install add. utils for docker (on RedHat systems)
sudo yum install -y yum-utils
Install docker
sudo yum install docker-ce docker-ce-cli containerd.io
Start docker engine
sudo systemctl start docker
Check status of docker
systemctl status docker
Get information about the docker installation
docker info
Container management
Create a container
docker create <image name>
Create a container and run it
docker run <image name>
Starting the given container
docker start <container name>
Stopping the given container (graceful)
docker stop <container name>
Restart (stop + start) the given container
docker restart <container name>
Kill the given container (SIGKILL)
docker kill <container name>
Pause the given container
docker pause <container name>
Resume the given container
docker unpause <container name>
Remove the container
docker rm <container name>
Force the removal of running container
docker rm -f <container name>
Real-time events from the given container
docker events <container-name>
Image management:
View docker images on local machine
docker images
Check running container
docker ps
Check all containers (running and stopped)
docker ps -a
Run the given container in background
docker run -d <container name>
Delete the container with the ID
docker rm <container id>
Show image history
docker history <image id>
Tag an image
docker tag <source image> <target image>
Delete an image
docker rmi <image name>
Get low-level information
docker inspect <image name>
Images
Pull an image from a Registry
docker pull <image name>
Push an image or repo to a registry
docker push <repository address> <image name>
Search for an image on docker hub
docker search <image name>
Load image from a tar archive
docker load <image tar file>
Save one image or more to a tar archive
docker save <file name> <options>
Login with Docker ID at Docker Hub
docker login
Build
Create an image from a dockerfile
docker build <options> <path to dockerfile>
-t <image name>
Run a command in a new container
docker run <options> <image name> <command>
Create a new container
docker create <Image name> <options> <command>
Create images using a git remote repository
docker git <git repository address>
Create a container with a custom name
docker container create -ti --name <container name> <image name>
Inspecting / Interacting
Show information about a container
docker inspect <container name>
List the processes inside the container
docker top <container name> <options>
List the logfile of the container
docker logs <container name>
Run a command in an existing container
docker exec <container name> <command>
Export a container to tar archive
docker export <container name>
Attach to a running container
docker attach <container name>
Copy files from the given container
docker cp <container name:path> hostpath\-
Copy files to the given container
docker cp hostpath\-<container name:path>
Get live stream statistics of given container
docker stats <container name>
List the installed plugins of docker
docker plugin ls
Clean up
Remove unused images
docker image prune
Remove all images
docker image prune -a
Prune your entire system
docker system prune
Kill all running containers
docker kill $(docker ps -q)
Author: Ralf Menti